Recently, I try to invest in P2P lending that helps provide funds to farmers. Though I often can't fund the project because the project itself is always funded really quickly. I complained about it to my friend and he replied why not create a cron to check it and notify you when new projects are posted. Oh, that's a good idea!
At first, I think that I must create a bot to posts the messages to the channel but it turns out Discord has a webhook to post messages to a specific channel. You still need admin access though to create the webhook.
Creating The Webhook
We will need to create a webhook URL that we can get from channel settings where we want to send our messages. You will need admin access to create the webhooks.
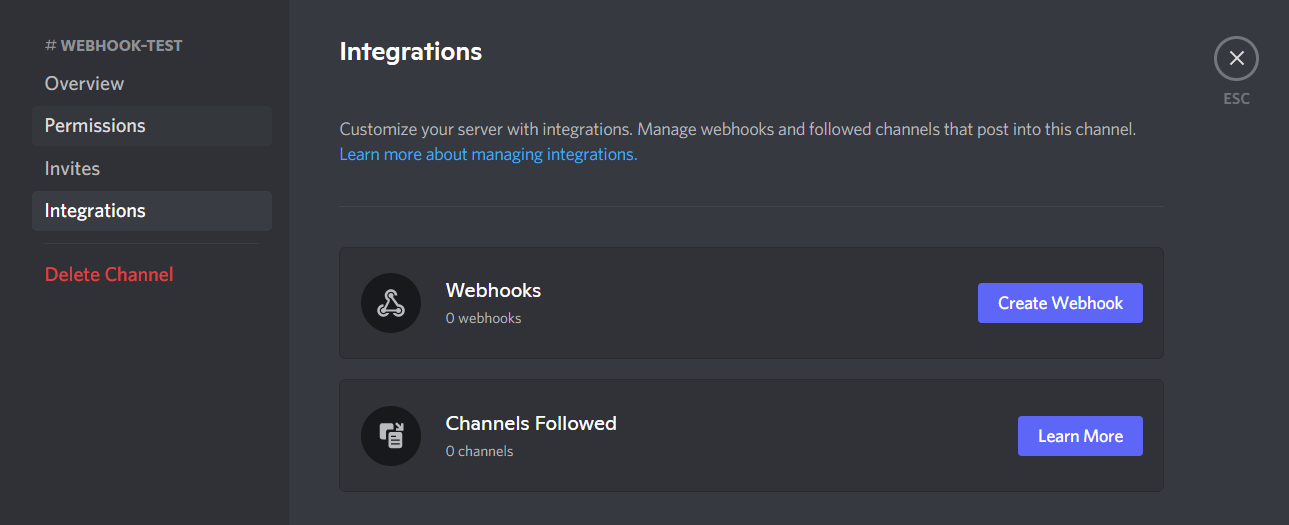
You can set your avatar and name when the webhook posts messages. Get the webhook URL from Copy Webhook URL
button.
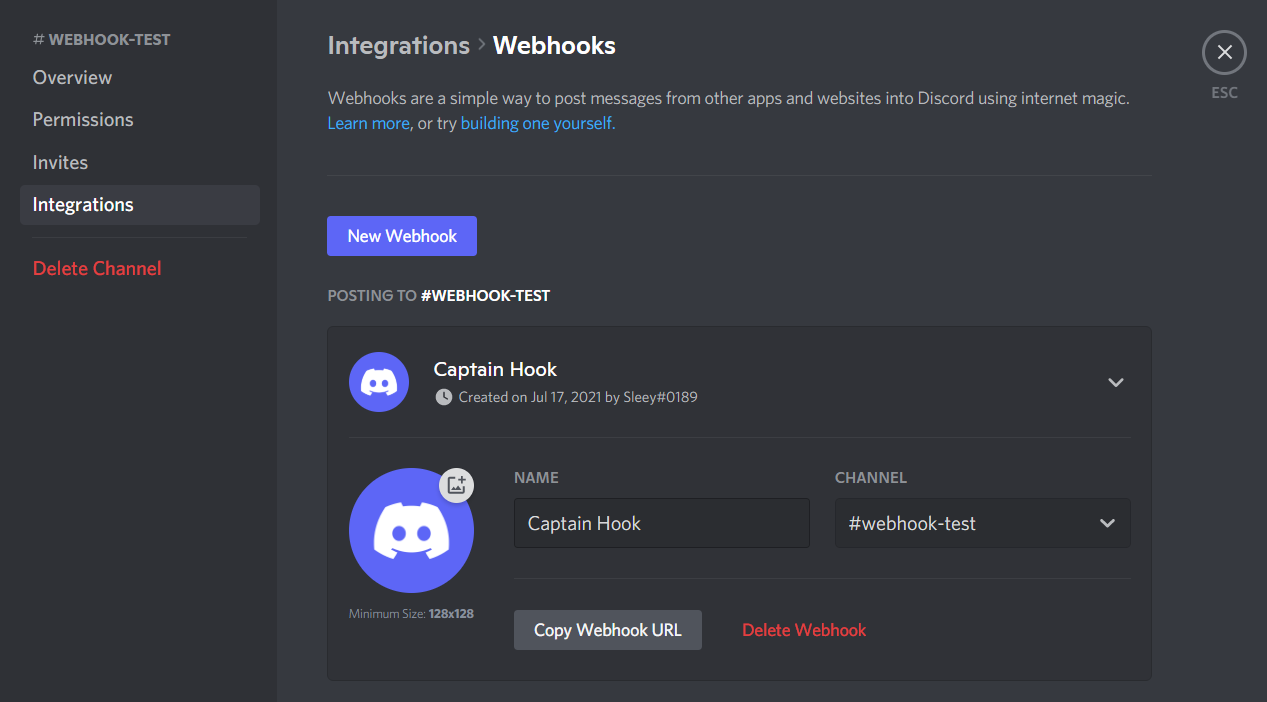
I want to finish the code as soon as possible because I don't want to spend a lot of time on this simple problem.
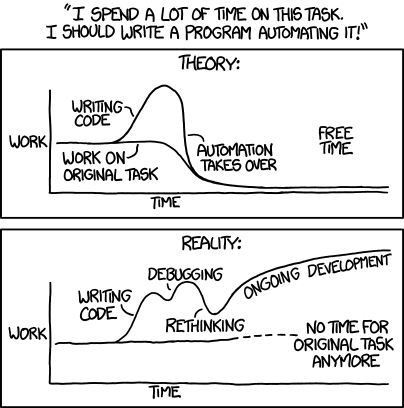
After reading the Discord webhook documentation, it's just a simple HTTP POST call. My programming language of choice for this is Python because it is perfect for scripting.
import requests
def sendMessageToDiscord(message):
url = "https://discord.com/api/webhooks/.../put_your_webhook_key_here"
data = {
"content": "<@123456789>\n" + message,
"username": "Paimon",
}
result = requests.post(url, json=data)
if 209 <= result.status_code < 300:
print("Success with {0}".format(result.status_code))
else:
print("Failed to sent message status code: {0}\nresponse:\n{1}".format(result.status_code,result.json()))
sendMessageToDiscord("Here is a message")
Or if you want to test it first using curl
.
curl --header "Content-Type: application/json" \
--request POST \
--data '{"content": "<@123456789>\nHere is a message", "username": "Paimon" }' \
https://discord.com/api/webhooks/.../put_your_webhook_key_here
The <@123456789>
is a user ID to be mentioned. So the above code will post a message like this in the channel.
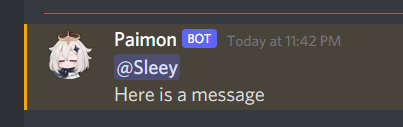
Creating The Cron
import time
import random
def getNewProjects():
# get P2P API and process the data to get the new project
message = getProjectList()
sendMessageToDiscord(message)
while True:
getNewProjects()
rand = random.randint(45,90)
time.sleep(rand)
print("Done waiting {0}s with last update {1}".format(rand, lastUpdate))
Another way will be to set it on the crontab if you just need a fixed interval. That's it, now you can send messages to Discord via webhook easily.